Simulate noise#
Objects from thztools
: NoiseModel
, NoiseModel.noise_amp
, NoiseModel.noise_sim
, timebase
, wave
.
[1]:
from matplotlib import pyplot as plt
import numpy as np
import thztools as thz
Set the simulation parameters#
[2]:
n = 256 # Number of samples
dt = 0.05 # Sampling time [ps]
sigma_alpha = 1e-4 # Additive noise amplitude [signal units]
sigma_beta = 1e-2 # Multiplicative noise amplitude [dimensionless]
sigma_tau = 1e-3 # Time base noise amplitude [ps]
Simulate a waveform#
Use wave
to simulate a terahertz time-domain waveform mu
with a timebase given by timebase
.
[3]:
t = thz.timebase(n, dt=dt)
mu = thz.wave(n, dt=dt)
Simulate noise#
Create an instance noise_model
of the NoiseModel
class and use the noise_sim
method to use the model to generate time-domain noise delta
for the waveform mu
.
[4]:
noise_model = thz.NoiseModel(sigma_alpha, sigma_beta, sigma_tau, dt=dt)
delta = noise_model.noise_sim(mu, seed=0)
sigma_mu = noise_model.noise_amp(mu)
Show the noise#
Show the simulated noise delta
together with statistical bounds given by the noise amplitude sigma_mu
.
[5]:
_, ax = plt.subplots()
ax.plot(t, delta)
ax.plot(t, sigma_mu, 'k:')
ax.plot(t, -sigma_mu, 'k:')
ax.set_xlabel('Time (ps)')
ax.set_ylabel(r'Amplitude (units of $\mu_{p})$')
ax.set_xticks(np.arange(0, 11, 5))
ax.set_xlim(0, 10)
plt.show()
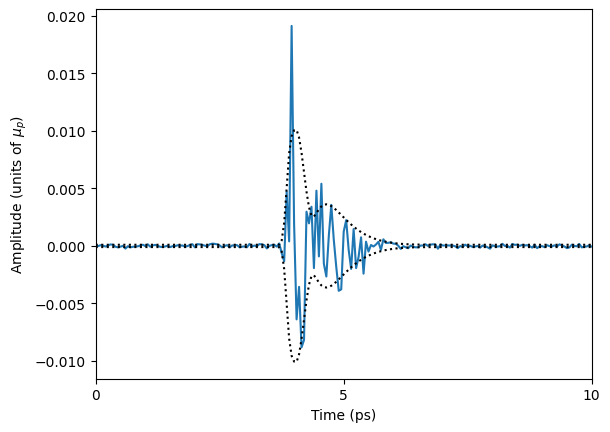